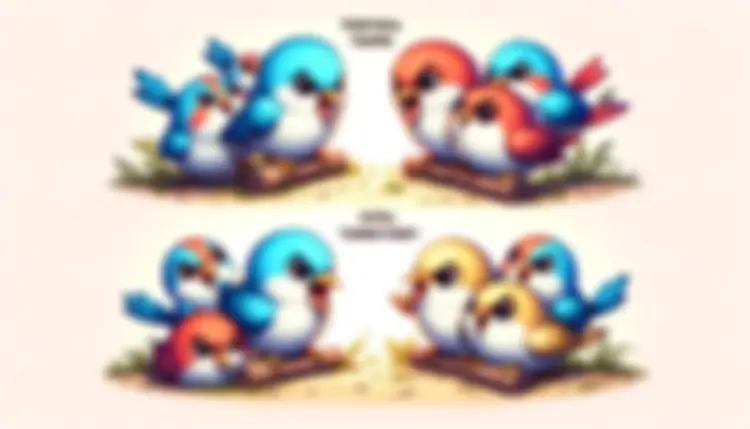
- Published: May 30, 2024
- Updated: Jul 8, 2025
<code style="color: red;">Unit</code> Although is one of the most frequently used types in Kotlin, its presence is surprisingly thin. For many programmers who have moved from Java to Kotlin, it may seem strange why Kotlin introduced the voidnew concept of instead of Java types . In fact, it is possible to develop Kotlin without any problems even if you do not fully understand . However, is closely related to Kotlin's grammar design and plays a quietly important role in Kotlin development.
What is a Unit?
The Importance of Meeting Delivery Dates
<code style="color: red">Unit</code> The most direct way to understand is to read the source code.
package kotlin
/**
* The type with only one value: the `Unit` object. This type corresponds
* to the `void` type in Java.
*/
public object Unit {
override fun toString() = "kotlin.Unit"
}
This <code style="color: red">Unit</code> is the source code of . As we can see from the source code, <code style="color: red">Unit</code> is a Kotlin class, or a Singleton Object.
Uses of Unit
Unitis an officially defined concept that is used as a return value when no value needs to be returned , to indicate that a function does not return any meaningful value. It is similar to Java's <code style="color: red">void</code>
Unit and void
Kotlin does not have truly no-return functions . The return type of a function that appears to have no return value is actually <code style="color: red">Unit</code>: It appears to have no return value because Kotlin automatically completes the omitted part.
For example, the following two code snippets are exactly the same, and the return type <code style="color: red">Unit</code> and return <code style="color: red">Unit</code> object can be omitted:
fun main() {
println("Hello world!")
}
fun main(): Unit {
println("Hello world!")
return Unit
}
<code style="color: red">Unit</code> This <code style="color: red">void</code> is the essential difference between Kotlin and Java.
Why is it designed this way?
The advantage of designing it this way <code style="color: red">void</code> is that it doesn't need to be treated specially. For example, your Java code might look like this:
abstract class Base<T> {
public abstract T make();
}
class Impl extends Base<Result> {
@Override
public Result make() {
return new Result();
}
}
For a class <code style="color: red">Base</code> that inherits from , like the one below , the method "does not need to return any value", but due to the limitations of inheritance and Generics, it is necessary to introduce methods different from and , and the method is implemented using the classes and .<code style="color: red">Nil</code> <code style="color: red">make</code> <code style="color: red">void</code> <code style="color: red">Void</code> <code style="color: red">return</code> <code style="color: red">null</code>
class Nil extends Base<Void> {
@Override
public Void make() {
return null;
}
}
In Kotlin, on the other hand, they are handled in the same way as regular functions, eliminating the need to introduce an additional concept.
internal class Nil : Base<Unit>() {
override fun make() {}
}
fun run(block: () -> Any) { TODO() }
run { "String" } // () -> String
run { 0 } // () -> Int
run {} // () -> Unit
Everything is an Expression
Like languages such as Python and Scala, the Kotlin language is based on the design philosophy of "Everything is an Expression", which <code style="color: red">void</code> is also the fundamental reason why Kotlin eliminates type specificity.
The "Everything is an Expression" design philosophy <code style="color: red">void</code> is also applied in other areas, such as:
- The return value is <code style="color: red">if-else</code> a statement
fun run(flag: Boolean) {
val value = if (flag) "Kotlin" else "Java"
println("Hello $value!")
}
fun run(flag: Int) {
val value = if (flag == 0) {
"Kotlin"
} else if (flag > 0) {
"Java"
} else {
"?"
}
println("Hello $value!")
}
- There is a return value <code style="color: red">when expression</code>
fun run(flag: Boolean) {
val value = when(flag) {
true -> "Kotlin"
else -> "Java"
}
println("Hello $value!")
}
fun run(flag: Int) {
val value = when {
flag == 0 -> "Kotlin"
flag > 0 -> "Java"
else -> "?"
}
println("Hello $value!")
}
Summary
In Kotlin, <code style="color: red">Unit</code> types play an important role, dictating that functions do not return meaningful values. <code style="color: red">Unit</code>By using types consistently, Kotlin <code style="color: red">void</code> removes the specialness of , making function signatures more uniform and making code more concise and clear.